You can create a new dropdown under the entity type ("Paragraphs types") that groups the links by first letter. Also, you can can change the weight of your item, and even move it out of being nested under configuration.
In the following example, we look for all the children of 'entity.paragraphs_type.collection' and we nest them under a copy of 'entity.paragraphs_type.collection' labeled with the first letter of the paragraphs underneath it.
function mymodule_menu_links_discovered_alter(&$links) {
$links['entity.paragraphs_type.collection']['weight'] = 8;
$links['entity.paragraphs_type.collection']['parent'] = 'system.admin';
foreach($links as $key => $link) {
if (!empty($link['parent']) && $link['parent'] == 'entity.paragraphs_type.collection') {
$first_letter = substr($link['title']->render(),0, 1);
$links['entity.paragraphs_type.collection.' . $first_letter] = [
'title' => $first_letter,
'route_name' => 'entity.paragraphs_type.collection',
'menu_name' => 'admin',
'parent' => 'entity.paragraphs_type.collection'
];
$links[$key]['parent'] = 'entity.paragraphs_type.collection.' . $first_letter;
}
}
}
Here's the same alphabetical grouping technique on vocabularies:
foreach($links as $key => $link) {
if (!empty($link['parent']) && $link['parent'] == 'entity.taxonomy_vocabulary.collection') {
$first_letter = substr($link['title']->render(),0, 1);
$links['entity.taxonomy_vocabulary.collection.' . $first_letter] = [
'title' => $first_letter,
'route_name' => 'entity.taxonomy_vocabulary.collection',
'menu_name' => 'admin',
'parent' => 'entity.taxonomy_vocabulary.collection'
];
$links[$key]['parent'] = 'entity.taxonomy_vocabulary.collection.' . $first_letter;
}
}
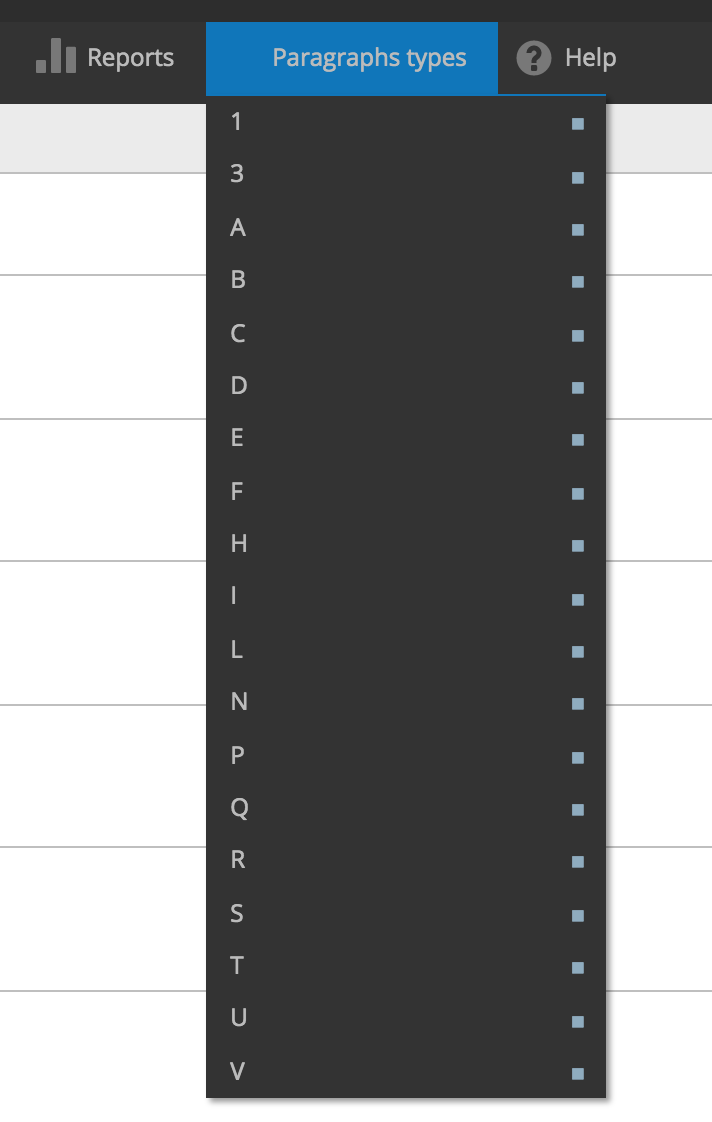
If you move the paragraph types to it's own drop-down, as in the example above, you'll want to style an icon for it.
.toolbar-icon-entity-paragraphs-type-collection:before,
.toolbar-icon-entity-paragraphs-type-collection:active:before,
.toolbar-icon-entity-paragraphs-type-collection.is-active:before {
background-image: url(../../../../core/themes/stable/images/core/icons/787878/wrench.svg);
}
Here's my current version, which adds an "Add Paragraph Type" link:
/**
* Implements hook_menu_links_discovered_alter().
*/
function mymodule_menu_links_discovered_alter(&$links) {
// Move paragraphs to its own drop down.
$links['entity.paragraphs_type.collection']['weight'] = -7;
$links['entity.paragraphs_type.collection']['parent'] = 'system.admin';
$links['entity.paragraphs_type.collection']['title'] = 'Paragraphs';
// Move taxonomy to its own drop down.
$links['entity.taxonomy_vocabulary.collection']['weight'] = -7;
$links['entity.taxonomy_vocabulary.collection']['parent'] = 'system.admin';
$links['entity.taxonomy_vocabulary.collection']['title'] = 'Taxonomy';
$links['entity.paragraphs_type.collection.add'] = [
'title' => new TranslatableMarkup('Add Paragraph Type'),
'route_name' => 'paragraphs.type_add',
'menu_name' => 'admin',
'parent' => 'entity.paragraphs_type.collection',
'weight' => -100,
];
// Break paragraphs into groups by starting letter.
foreach ($links as $key => $link) {
if (!empty($link['parent']) && $link['parent'] == 'entity.paragraphs_type.collection') {
$first_letter = substr($link['title']->render(), 0, 1);
$links['entity.paragraphs_type.collection.' . $first_letter] = [
'title' => $first_letter,
'route_name' => 'entity.paragraphs_type.collection',
'menu_name' => 'admin',
'parent' => 'entity.paragraphs_type.collection',
];
$links[$key]['parent'] = 'entity.paragraphs_type.collection.' . $first_letter;
}
}
foreach ($links as $key => $link) {
if (!empty($link['parent']) && $link['parent'] == 'entity.taxonomy_vocabulary.collection') {
$first_letter = substr($link['title']->render(), 0, 1);
$links['entity.taxonomy_vocabulary.collection.' . $first_letter] = [
'title' => $first_letter,
'route_name' => 'entity.taxonomy_vocabulary.collection',
'menu_name' => 'admin',
'parent' => 'entity.taxonomy_vocabulary.collection',
];
$links[$key]['parent'] = 'entity.taxonomy_vocabulary.collection.' . $first_letter;
}
}
}